Use Case description
As a device manufacturer or firmware developer, I would like my devices to automatically provision themselves in ThingsBoard. During the automatic provisioning, the device may either generate unique credentials or ask the server to provide unique credentials for the device.
From the 3.5 version, ThingsBoard allows the auto-provision of new devices on authentication over MQTT using X.509 Certificate chain.
How it works?
The device may send a device provisioning request (Request) to the ThingsBoard. The Request should always contain a Provision key and secret. The Request may optionally include the device name and credentials generated by the device. If those credentials are absent, the Server will generate an Access Token to be used by the device.
Provisioning request example:
1
2
3
4
5
{
"deviceName": "DEVICE_NAME",
"provisionDeviceKey": "YOUR_PROVISION_KEY_HERE",
"provisionDeviceSecret": "YOUR_PROVISION_SECRET_HERE"
}
The ThingsBoard validates the Request and replies with the device provisioning response (Response). The successful response contains device id, credentials type, and body. If the validation was not successful, the Response will contain only the status.
Provisioning response example:
1
2
3
4
5
{
"provisionDeviceStatus":"SUCCESS",
"credentialsType":"ACCESS_TOKEN",
"accessToken":"sLzc0gDAZPkGMzFVTyUY"
}
During the validation of the Request, ThingsBoard will first check the supplied provisionDeviceKey and provisionDeviceSecret to find the corresponding Device Profile. Once the profile is found, the platform will use the configured provision strategy to validate the device name. There are two provision strategies available:
- Allowing creating new devices - checks that the device with the same name has not registered in ThingsBoard yet. This strategy is useful when you don’t know the list of unique device names (MAC addresses, etc.) during manufacturing, but the device itself has access to this info in the firmware. It is easier to implement, but it is less secure than the second strategy.
- Checking pre-provisioned devices - checks that the device with the same name has been already created in ThingsBoard, but hasn’t been provisioned yet. This strategy is useful when you want to allow provisioning only for a specific list of devices. Let’s assume that you have collected a list of unique IDS (MAC addresses, etc) during the manufacturing. You can use bulk provisioning to upload this list to ThingsBoard. Now, devices from the list can issue provision requests, and no other devices will be able to provision themselves.
Once the provisioning is complete, ThingsBoard will update provisionState server attribute of the device and will set it to provisioned value.
Device profile configuration
You should configure the device profile to enable provisioning feature, collect provision device key and provision device secret.
- You can either create a new device profile or open the existing one. To create a new one you should open the Device profiles page and click on the "+" icon in the table header.
- Input a name of the new device profile and click on Step 4 of the "Add device profile" wizard. We will use name "Device Provisioning Test" in this example. However, typically this should be your device model or similar.
- Choose one of the provisioning strategies, copy the provisioning key and secret, and finally click "Add".
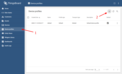
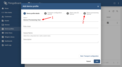
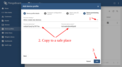
Provision Device APIs
MQTT Device APIs
You can use the MQTT API reference to develop your device firmware that will perform the provision request. As mentioned earlier, a device can request the server to generate the credentials or to provide its own credentials during the registration process. See request/response and code examples for each option below:
Provisioning request data example:
Provisioning response example:
Sample scriptTo communicate with ThingsBoard we will use Paho MQTT module, so we should install it:
The script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Provisioning request data example:
Provisioning response example:
Sample scriptTo communicate with ThingsBoard we will use Paho MQTT module, so we should install it:
The script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Provisioning request data example:
Provisioning response example:
Sample scriptTo communicate with ThingsBoard we will use Paho MQTT module, so we should install it:
The script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Provisioning request data example:
Provisioning response example:
MQTT Example scriptTo use this script put your mqttserver.pub.pem (public key of the server) into the folder with script.
|
HTTP Device APIs
You may use the HTTP API reference to develop your device firmware that will perform the provision request. As mentioned earlier, the device may request server to generate the credentials or provide its own credentials during the registration process. See request/response and code examples for each option below:
Provisioning request data example:
Provisioning response example:
Sample scriptThe script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Provisioning request data example:
Provisioning response example:
Sample scriptThe script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
CoAP Device APIs
You may use the CoAP API reference to develop your device firmware that will perform the provision request.
As mentioned earlier, the device may request server to generate the credentials or provide its own credentials during the registration process.
See request/response and code examples for each option below:
Provisioning request data example:
Provisioning response example:
Sample scriptTo communicate with ThingsBoard we will use asyncio and aiocoap modules, so we should install it:
The script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Provisioning request data example:
Provisioning response example:
Sample scriptTo communicate with ThingsBoard we will use asyncio and aiocoap modules, so we should install it:
The script source code is available below. You may copy-paste it to a file, for example:
Now you should run the script and follow the steps inside.
The script source code:
|
Next steps
-
Getting started guides - These guides provide quick overview of main ThingsBoard features. Designed to be completed in 15-30 minutes.
-
Installation guides - Learn how to set up ThingsBoard on various available operating systems.
-
Connect your device - Learn how to connect devices based on your connectivity technology or solution.
-
Data visualization - These guides contain instructions on how to configure complex ThingsBoard dashboards.
-
Data processing & actions - Learn how to use ThingsBoard Rule Engine.
-
IoT Data analytics - Learn how to use rule engine to perform basic analytics tasks.
-
Hardware samples - Learn how to connect various hardware platforms to ThingsBoard.