- Enable Custom prediction model
- Define input dataset
- Basic univariable python model example
- Multivariable python model example
- Next Steps
You can add new prediction models into Trendz by writing a custom Python code. This code will be executed on the server side and will have access to the whole input dataset that includes required telemetries and attributes data. You can import required Python libraries and use them in your code to forecast required metric based on input data.
Enable Custom prediction model
Once you added required telemetry or calculated fields into Trendz view, you can tell Trendz that it should use custom prediction model for this field.
To do that you need to open Field settings
dialog and select Custom
option in the Prediction method
dropdown:
Define input dataset
By default, you will have only original telemetry data in the input dataset. But you can add additional telemetries and attributes into the input dataset by selecting them in Selected fields for prediction
section.
Just start typing the name of telemetry or attribute and select required field from the dropdown list.
Basic univariable python model example
In this example we will show how to predict water consumption based on historical data.
- Create Bar chart view in Trendz
- Add
Date
field into X-axis section - Add
consumption
telemetry into the Y-axis section - Add
Sensor
device name into filter section and select required device - Enable
Prediction
checkbox and selectCustom
option in thePrediction method
dropdown - Set prediction time range for next 14 days
- Write following code into the
Model function
section:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
import pandas as pd
from prophet import Prophet
print(f"inputX: {inputX}")
print(f"inputY: {inputY}")
print(f"outputX: {outputX}")
df = pd.DataFrame()
df['ds'] = pd.to_datetime(inputX, unit='ms')
df['y'] = inputY
model = Prophet()
model.fit(df)
future = pd.DataFrame()
future['ds'] = pd.to_datetime(outputX, unit='ms')
forecast = model.predict(future)
outputY = forecast['yhat'].tolist()
print(f"result: {outputY}")
return outputY
Now you can build view to see the result of your prediction model.
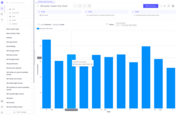
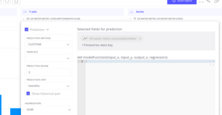
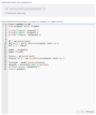
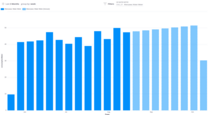
Multivariable python model example
In this example we will show how to predict heat consumption based on historical consumption, environment temperature .
- Create Bar chart view in Trendz
- Add
Date
field into X-axis section - Add
consumption
telemetry into the Y-axis section - Add
Sensor
device name into filter section and select required device - Enable
Prediction
checkbox and selectCustom
option in thePrediction method
dropdown - Set prediction time range for next 14 days
- Add
temperature
field into Selected fields for prediction - Write following code into the
Model function
section:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
import pandas as pd
import numpy as np
from prophet import Prophet
futureRegressors = []
regressorsCount = len(historyRegressors)
for i in range(0, regressorsCount):
regressorInputX = inputX
regressorOutputX = outputX
regressorInputY = historyRegressors[i]
regressorOutputY = []
df = pd.DataFrame()
df['ds'] = pd.to_datetime(regressorInputX, unit='ms')
df['y'] = regressorInputY
model = Prophet()
model.fit(df)
future = pd.DataFrame()
future['ds'] = pd.to_datetime(regressorOutputX, unit='ms')
forecast = model.predict(future)
regressorOutputY = forecast['yhat'].tolist()
futureRegressors.append(regressorOutputY)
for i in range(0, regressorsCount):
print(f"historyRegressors{i} = {historyRegressors[i]}")
for i in range(0, regressorsCount):
print(f"futureRegressors{i} = {futureRegressors[i]}")
print(f"inputX: {inputX}")
print(f"inputY: {inputY}")
print(f"outputX: {outputX}")
df = pd.DataFrame()
df['ds'] = pd.to_datetime(inputX, unit='ms')
df['y'] = np.array(inputY)
for i in range(0, regressorsCount):
df['regressor' + str(i)] = np.array(historyRegressors[i])
model = Prophet()
for i in range(0, regressorsCount):
model.add_regressor('regressor' + str(i), standardize=False)
model.fit(df)
future = pd.DataFrame()
future['ds'] = pd.to_datetime(outputX, unit='ms')
for i in range(0, regressorsCount):
future['regressor' + str(i)] = np.array(futureRegressors[i])
forecast = model.predict(future)
outputY = forecast['yhat'].tolist()
print(f"result: {outputY}")
return outputY
Next Steps
-
Getting started guide - These guide provide quick overview of main Trendz features.
-
Installation guides - Learn how to setup ThingsBoard on various available operating systems.
-
States - Learn how to define and analyse states for assets based on raw telemetry.
-
Group and Aggregate data - Learn how to group and aggregate data in Trendz.
-
Prediction - Learn how to make forecasts and predict telemetry behavior.
-
Filters - Learn how filter dataset during analysis.
-
Available Visualizations - Learn about visualization widgets available in Trendz and how to configure them.
-
Share and embed Visualizations - Learn how to add Trendz visualizations on ThingsBoard dashboard or 3rd party web pages.